Reduce boilerplate with ChakraUI and NextJS
What is ChakraUI
ChakraUI (and the future version which i prefer- https://next.chakra-ui.com/ ) is a React UI framework built by Segun Adebayo that has a different approach to composing user interfaces.
It views accessibility and modularity as a first-class citizen, and is inspired by the TailwindCSS utility based css framework.
What I like about it the most is that you can built a whole application without actually writing any CSS yourself and with using the utilities ChakraUI provides.
An example from the ChakraUI homepage:
Which would render the following UI:
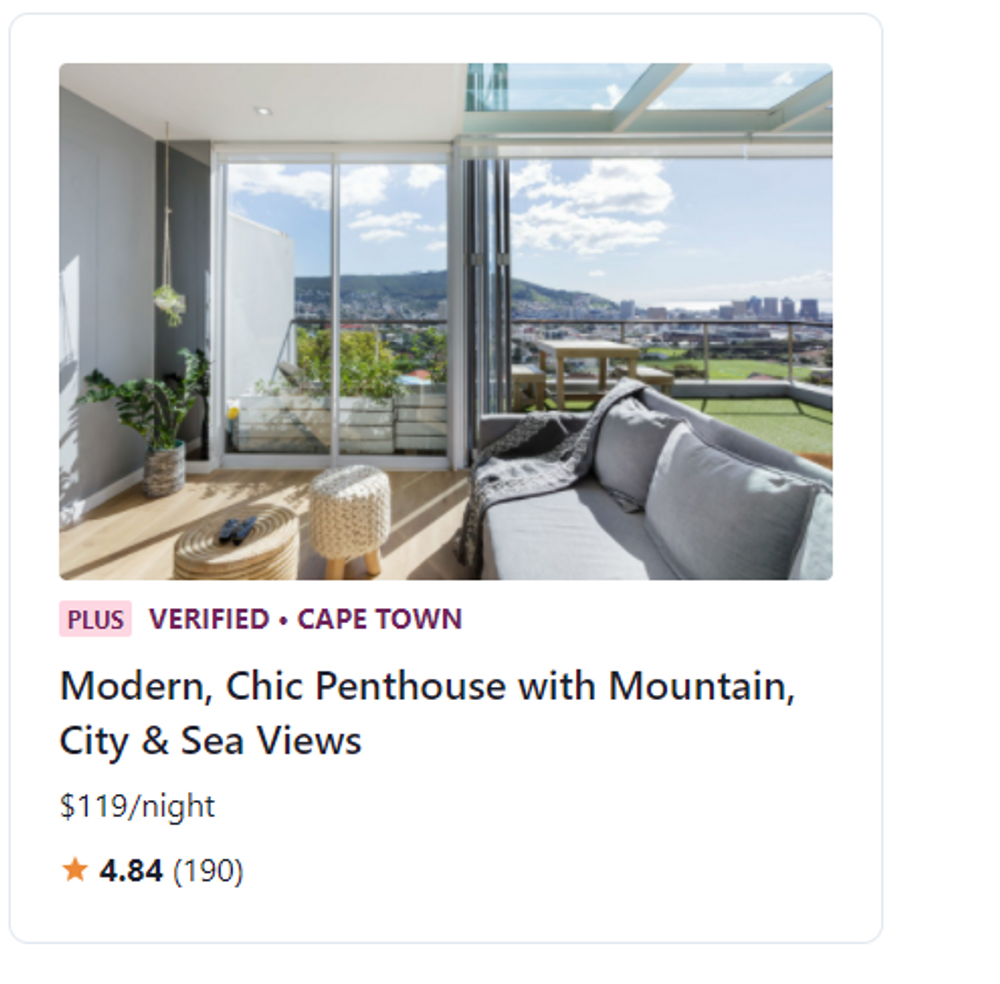
As you can see, it is very easy to build custom UI components with Chakra.
What is Next.js?
Put simply - Next.js is a superpowered React application framework.
It provides many out-of-the-box features you would usually need to install into a pure react app and configure yourself:
- Server-Side Rendering
- Static rendering
- Routing and route pre-fetching
- Smart bundling
- API Endpoints
And all that just by creating a blank Next.js app and without additional configuration.
Additionally, it is easy to get started just by following their step by step tutorial - https://nextjs.org/learn/basics/create-nextjs-app
Connecting them together
The first thing you need to do is add the Chakra context provider around your Next.js pages.
I will be using examples from my latest web project - Stackpal.me which I've build with exactly ChakraUI and Next.js.
If you don't have an _app.js file in your "pages" folder, create it now with the following contents:
You can also see a simple example on how to add theming to ChakraUI in a single place.
The brand colors are added directly to the provider and can be used in the whole application by just using the color name, for example "brand.50" would be #004d4e.
This makes it very easy to change your brand colors at any time.
By adding te ChakraProvider around all of our pages, we have enabled the use of all the ChakraUI components anywhere in the app.
For example, the forgotten password button is simple this:
One other interesting thing you can see here is the useColorModeValue hook.
ChakraUI comes with dark/light theme out of the box and provides this hook that returns a color mode aware value.
To enable color mode, we need to add a _document.js file to our Next.js app. If you don't have it already, create one in the pages directory with the following contents:
You can see I've provided the "light" color mode as a default, but this can also be "dark", or can automatically be detected from the system color scheme - (more details: https://next.chakra-ui.com/docs/features/color-mode#change-color-mode-behavior)
Chakra also provides the useColorMode hook which can be used to toggle the color mode from any part of the app:
Simply calling "toggleColorMode()" from a button would switch the whole app to dark mode.
What is missing
Of course, no solution is perfect and there is always a slice of the pie missing.
To make this a complete app framework, we need who more things for which I will propose solutions.
A backend
Your application data needs to be stored somewhere, I would suggest Firebase with the amazing useFirestoreQuery hook from Gabe Ragland.
State management
No react app is complete with some kind of over-complicated state management framework. But it doesn't have to be overcomplicated.
The newest state management library build at Facebook itself is Recoil and it greatly simplifies global state management. Make sure to give it a try.
Your feedback
Disagree with something, want to talk about this blog post?
Feel free to contact me at any time on Twitter: @TheEskhaton1